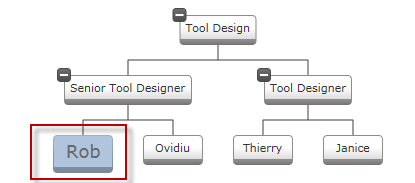
Provides detailed instructions, complete with code examples, on how to apply conditional formatting to xamOrgChart ™ nodes
Prerequisite topics required to understand this material.
This topic contains the following sections:
Conditional formatting is a method of customizing the appearance of individual or groups of nodes within a node layout. The appearance of the node may include the style, color, font family and size, the foreground or background of the node.
For example, say you have defined conditional formatting in your application based on a data value. If the data value meets the specified condition, say “Account Balance” below zero display the node’s text in Red Italics . Whenever Account Balance falls below zero xamOrgChart renders the node in accordance with the conditional formatting, in this case Red Italics .
Since conditional formatting is an optional feature, if no conditions are specified then the applied format and style is applied to all nodes of the control. When working with conditional formatting, it is important to note that while the style can be created in XAML and referenced as a resource the underlying conditional logic can only be implemented in code using C# or Visual Basic. This is because the nodes in the xamOrgChart control are represented by OrgChartNode objects, which can be referenced in code (C# or Visual Basic), but not in XAML. Consequently, conditional formatting can only be achieved in code.
Because the xamOrgChart control does not expose the node objects in XAML, all conditional formatting must be implemented in either C# or Visual Basic code.
Following is a conceptual overview of the process:
1. Defining a style for the conditional formatting
2. Verifying the condition and applying the formatting by handling the NodeControlAttachedEvent
The following example illustrates how conditional formatting can be applied to a particular employee in an employee database; causing him or her to stand out in the xamOrgChart layout.
This example employed XAML to create the underlying style with a specific formatting of foreground, background, and font size properties. The conditional logic, in this case, was set up to identify an individual employee (by first name) out of an employee database and apply the style that was defined in XAML. This can only be implemented in code using either C# or Visual Basic.
Here, we can see how the conditional formatting is applied to a sub node in the tree, in this case Rob.
To complete this procedure, you will need the following:
A WPF or Silverlight project with a Window (WPF) or UserControl (Silverlight) added to it
A xamOrgChart control added to the page. See Adding xamOrgChart to Your Application for information on adding and binding a control to data.
This topic takes you step-by-step through the process of conditional formatting. The following is a conceptual overview of the process:
1. Defining a style for the conditional formatting
2. Verifying the condition and applying the formatting by handling the NodeControlAttachedEvent
To see how easy it is to apply conditional formatting to your application, follow these steps in order.
Define the style for condition formatting In XAML as follows:
In XAML:
<Style x:Key="EmployeeNodeStyle"
TargetType="ig:OrgChartNodeControl">
<Setter Property="Background" Value="#FFB0C4DE"/>
<Setter Property="Foreground" Value="#FF696969" />
<Setter Property="FontSize" Value="18" />
</Style>
In code behind, add the following code for conditional formatting.
The code performs the following steps:
It retrieves and validates the data.
It verifies that the object type is the correct type.
It applies the XAML formatted style created earlier.
In C#:
private void XamOrgChart_NodeControlAttachedEvent(object sender, Infragistics.Controls. Maps.OrgChartNodeEventArgs e)
{
var nodeModel = e.Node.DataContext;
if (nodeModel == null)
return;
if (nodeModel.GetType() == typeof(Employee))
{
Employee emp = nodeModel as Employee;
if (emp == null)
return;
if ((string)emp.FirstName == "Rob")
{
e.Node.Style = this.Resources["EmployeeNodeStyle"] as Style;
}
}
}
In Visual Basic:
Private Sub XamOrgChart_NodeControlAttachedEvent(sender As Object, e As Infragistics.Controls.Maps.OrgChartNodeEventArgs)
Dim nodeModel = e.Node.DataContext
If nodeModel Is Nothing Then
Return
End If
If nodeModel.[GetType]() = GetType(Employee) Then
Dim emp As Employee = TryCast(nodeModel, Employee)
If emp Is Nothing Then
Return
End If
If DirectCast(emp.FirstName, String) = "Rob" Then
e.Node.Style = TryCast(Me.Resources("EmployeeNodeStyle"), Style)
End If
End If
End Sub