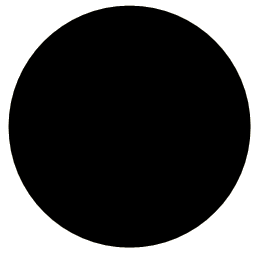
The Dial property determines the shape of a Radial gauge. By default, the dial sweeps 360 degrees, so it appears as a circle.
This topic guides you through the first step in creating a new Radial gauge. After completing the steps outlined in this topic, you will have created a new Radial gauge with the Dial property set to a solid black background.
The next step after creating a Dial is to Add a Scale to a Gauge.
You can configure the Dial property of your gauge:
When you save and run your application after completing the following steps, your gauge should look similar to the gauge below.
To configure the Dial property of your Radial gauge using the Gauge Designer:
In the Gauge Explorer, expand the Gauges property.
Click Add Gauge… and select New Radial Gauge.
Expand Radial Gauge and select Dial.
In the Appearance tab of the Properties panel, select Solid from the Type drop-down list.
Click the Details drop-down arrow.
The color picker appears. On the left-hand side, click Web, then select the Black color.
To configure the Dial property of your Radial gauge at design time:
In Design view, click the UltraGauge control on your form.
In the Properties window, click the Gauges property. Then, click the ellipsis button (…) to open the Gauges collection editor.
In the Gauges collection editor, click Add, and select Add Radial Gauge.
Expand the Dial property.
Create a new BrushElement object by clicking the BrushElement property. Then from the drop-down list, select Solid Fill.
Expand the BrushElement property, and set the Color property to Black.
To configure the Dial property of your Radial gauge at run time:
Before you start writing any code, you should place using/Imports directives in your code-behind so you don’t need to always type out a member’s fully qualified name.
In Visual Basic:
Imports Infragistics.UltraGauge.Resources
In C#:
using Infragistics.UltraGauge.Resources;
Create the load event.
Create a Gauge collection, a Radial gauge and the brush element:
In Visual Basic:
Dim ultraGauge1 As New Infragistics.Win.UltraWinGauge.UltraGauge() Dim myRadialGauge As New RadialGauge() Dim mySolidFillBrushElement As New SolidFillBrushElement()
In C#:
Infragistics.Win.UltraWinGauge.UltraGauge ultraGauge1 = new Infragistics.Win.UltraWinGauge.UltraGauge(); RadialGauge myRadialGauge = new RadialGauge(); SolidFillBrushElement mySolidFillBrushElement = new SolidFillBrushElement();
Set the following color properties:
Type — Solid
Color — Black
In Visual Basic:
mySolidFillBrushElement.Color = System.Drawing.Color.Black myRadialGauge.Dial.BrushElement = mySolidFillBrushElement
In C#:
mySolidFillBrushElement.Color = System.Drawing.Color.Black; myRadialGauge.Dial.BrushElement = mySolidFillBrushElement;
Add your Radial gauge to the gauges collection. Set the following properties:
Location — (30, 4)
Name — ultraGauge1
Size — (250, 250)
TabIndex — 0
In Visual Basic:
ultraGauge1.Gauges.Add(myRadialGauge) ultraGauge1.Location = New System.Drawing.Point(30, 4) ultraGauge1.Name ="ultraGauge1" ultraGauge1.Size = New System.Drawing.Size(250, 250) ultraGauge1.TabIndex = 0 Me.Controls.Add(ultraGauge1)
In C#:
ultraGauge1.Gauges.Add(myRadialGauge); ultraGauge1.Location = new System.Drawing.Point(30, 4); ultraGauge1.Name = "ultraGauge1"; ultraGauge1.Size = new System.Drawing.Size(250, 250); ultraGauge1.TabIndex = 0; this.Controls.Add(ultraGauge1);