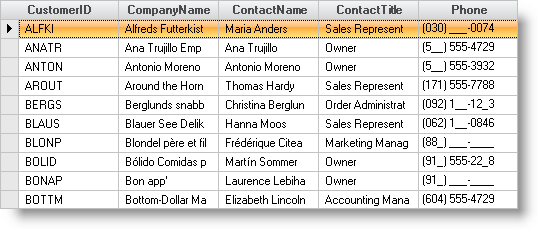
User input into cells which should contain a specific number of characters of input with each character being of a specific input type (ex: US style phone number "(316) 555-1212") are best formatted using the WinGrid™ MaskInput features.
How do I format a Phone number so that it makes the proper US style phone numbers?
User input prompting and formatting can be performed by setting the MaskInput property of the UltraWinGrid to a mask indicating the position of the literals and input characters.
Properties associated with the UltraWinGrid MaskInput feature include:
MaskClipMode — Returns or sets a value that determines how cell values for a column will be copied to the clipboard when data masking is in enabled.
MaskDataMode — Returns or sets a value that determines how cell values for a column will be stored by the data source when data masking is enabled.
MaskDisplayMode — Returns or sets a value that determines cell values will be displayed when the cells are not in edit mode and data masking is enabled.
MaskInput — Determines the input mask for the cell. Characters used to create the MaskInput are:
MaskLiteralsAppearance — If the cell has MaskInput set or is using a UltraMaskedEdit element then this appearance will be applied to the literal chars while in edit mode.
PadChar — Determines the pad character used for formatting text with mask.
PromptChar — Returns or sets a value that determines the prompt character used during masked input.
The "Phone" column is formatted with the mask "() -##".
UltraGrid1.InitializeLayout — The "Phone" column MaskInput property is set to the phone number mask. The MaskDataMode is set so the grid doesn’t try to put the formatting into the numeric database field. The MaskClipMode is set to IncludeBoth so when the user performs a copy operation on the cell the formatting is placed with the data on the clipboard. The MaskDisplayMode is set to IncludeBoth so the cell is displayed with the mask:
In Visual Basic:
Imports Infragistics.Win.UltraWinMaskedEdit ... Private Sub UltraGrid1_InitializeLayout(ByVal sender As Object, _ ByVal e As Infragistics.Win.UltraWinGrid.InitializeLayoutEventArgs) _ Handles UltraGrid1.InitializeLayout e.Layout.Bands(0).Columns("Phone").MaskInput = "(###) ###-####" e.Layout.Bands(0).Columns("Phone").MaskDataMode = MaskMode.Raw e.Layout.Bands(0).Columns("Phone").MaskClipMode = MaskMode.IncludeBoth e.Layout.Bands(0).Columns("Phone").MaskDisplayMode = MaskMode.IncludeBoth End Sub
In C#:
using Infragistics.Win.UltraWinMaskedEdit; ... private void ultraGrid1_InitializeLayout(object sender, Infragistics.Win.UltraWinGrid.InitializeLayoutEventArgs e) { e.Layout.Bands[0].Columns["Phone"].MaskInput = "(###) ###-####"; e.Layout.Bands[0].Columns["Phone"].MaskDataMode = MaskMode.Raw; e.Layout.Bands[0].Columns["Phone"].MaskClipMode = MaskMode.IncludeBoth; e.Layout.Bands[0].Columns["Phone"].MaskDisplayMode = MaskMode.IncludeBoth; }
This project illustrates the use of the Mask properties to format column information for display, clipboard, database and user input. It also illustrates the versatility of the Data, Clipboard and Display modes so that data can be displayed with or without formatting.