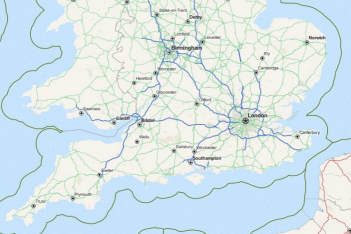
This topic provides information on how to create and display custom geographic imagery from Map Quest in the background content of the XamGeographicMap™ control.
The following table lists the topics required as a prerequisite to understanding this topic.
This topic contains the following sections:
The Map Quest geographic imagery is a free mapping service created by MapQuest® company. This geographic imagery service can be accessed directly on http://www.mapquest.com web site.
By the default, the XamGeographicMap control does not provide support for geographic imagery from the Map Quest service. However, the GeographicMapImagery class can be extended to provide implementation for geographic imagery tiles from other geographic imagery sources.
The following image is a preview of the XamGeographicMap control with geographic imagery tiles from the Map Quest service.
The following procedure demonstrates how to create and display geographic imagery from Map Quest in the background content of the XamGeographicMap control by extending the link:InfragisticsWPF.datavisualization~Infragistics.Controls.Maps.maptilesource_members.html [MapTileSource] and GeographicMapImagery classes.
Create multi-scale tile source for the Map Quests geographic imagery by inheriting MapTileSource class.
In Visual Basic:
Namespace IGGeographicMap.Extensions
''' <summary>
''' Represents tile source for geographic imagery from the Map Quest service
''' </summary>
Public Class MapQuestTileSource
Inherits Infragistics.Controls.Maps.MapTileSource
Public Sub New()
MyBase.New(134217728, 134217728, 256, 256, 0)
End Sub
#Region "TileMapStyle"
''' <summary>
''' Gets or sets map style for the tile source of MapQuest geographic imagery
''' </summary>
Public Property TileMapStyle() As MapQuestImageryStyle
Get
Return _TileMapStyle
End Get
Set
_TileMapStyle = Value
End Set
End Property
Private _TileMapStyle As MapQuestImageryStyle
#End Region
Private Const TileStreetPath As String = "http://otile4.mqcdn.com/tiles/1.0.0/osm/{Z}/{X}/{Y}.png"
Private Const TileAerialPath As String = "http://oatile3.mqcdn.com/naip/{Z}/{X}/{Y}.png"
''' <summary>
''' Gets path for the type of a geographic imagery tile
''' </summary>
''' <returns></returns>
Private Function GetTileType() As String
Return If(Me.TileMapStyle = MapQuestImageryStyle.SatelliteMapStyle, TileAerialPath, TileStreetPath)
End Function
''' <summary>
''' Overridden method for getting a geographic imagery tile at specific position of the map
''' </summary>
Protected Overrides Sub GetTileLayers(tileLevel As Integer, tilePositionX As Integer, tilePositionY As Integer, tileImageLayerSources As System.Collections.Generic.IList(Of Object))
Dim tilePath = GetTileType()
Dim zoom As Integer = tileLevel - 8
If zoom > 0 Then
Dim uriString As String = tilePath
uriString = uriString.Replace("{Z}", zoom.ToString())
uriString = uriString.Replace("{X}", tilePositionX.ToString())
uriString = uriString.Replace("{Y}", tilePositionY.ToString())
tileImageLayerSources.Add(New Uri(uriString))
End If
End Sub
End Class
''' <summary>
''' Defines style of the MapQuest geographic imagery.
''' </summary>
Public Enum MapQuestImageryStyle
''' <summary>
''' Specifies street style of geographic imagery from the Map Quest service
''' </summary>
StreetMapStyle
''' <summary>
''' Specifies satellite style of geographic imagery from the Map Quest service
''' </summary>
SatelliteMapStyle
End Enum
End Namespace
In C#:
using System;
namespace IGGeographicMap.Extensions
{
/// <summary>
/// Represents tile source for geographic imagery from the Map Quest service
/// </summary>
public class MapQuestTileSource : Infragistics.Controls.Maps.MapTileSource
{
public MapQuestTileSource()
: base(134217728, 134217728, 256, 256, 0)
{ }
#region TileMapStyle
/// <summary>
/// Gets or sets map style for the tile source of MapQuest geographic imagery
/// </summary>
public MapQuestImageryStyle TileMapStyle { get; set; }
#endregion
private const string TileStreetPath = "http://otile4.mqcdn.com/tiles/1.0.0/osm/{Z}/{X}/{Y}.png";
private const string TileAerialPath = "http://oatile3.mqcdn.com/naip/{Z}/{X}/{Y}.png";
/// <summary>
/// Gets path for the type of a geographic imagery tile
/// </summary>
/// <returns></returns>
private string GetTileType()
{
return this.TileMapStyle == MapQuestImageryStyle.SatelliteMapStyle ? TileAerialPath : TileStreetPath;
}
/// <summary>
/// Overridden method for getting a geographic imagery tile at specific position of the map
/// </summary>
protected override void GetTileLayers(int tileLevel, int tilePositionX, int tilePositionY, System.Collections.Generic.IList<object> tileImageLayerSources)
{
var tilePath = GetTileType();
int zoom = tileLevel - 8;
if (zoom > 0)
{
string uriString = tilePath;
uriString = uriString.Replace("{Z}", zoom.ToString());
uriString = uriString.Replace("{X}", tilePositionX.ToString());
uriString = uriString.Replace("{Y}", tilePositionY.ToString());
tileImageLayerSources.Add(new Uri(uriString));
}
}
}
/// <summary>
/// Defines style of the MapQuest geographic imagery.
/// </summary>
public enum MapQuestImageryStyle
{
/// <summary>
/// Specifies street style of geographic imagery from the Map Quest service
/// </summary>
StreetMapStyle,
/// <summary>
/// Specifies satellite style of geographic imagery from the Map Quest service
/// </summary>
SatelliteMapStyle,
}
}
Create geographic imagery classes for supported types of map styles of the Map Quests service by inheriting from the GeographicMapImagery class and providing Map Quests imagery source.
In Visual Basic:
Imports Infragistics.Controls.Maps
Namespace IGGeographicMap.Extensions
''' <summary>
''' Represents geographic imagery with street map style from the Map Quest service
''' </summary>
Public Class MapQuestStreetImagery
Inherits GeographicMapImagery
Public Sub New()
MyBase.New(New MapQuestTileSource() With { .TileMapStyle = MapQuestImageryStyle.StreetMapStyle })
End Sub
End Class
''' <summary>
''' Represents geographic imagery with satellite map style from the Map Quest service
''' </summary>
Public Class MapQuestSatelliteImagery
Inherits GeographicMapImagery
Public Sub New()
MyBase.New(New MapQuestTileSource() With { .TileMapStyle = MapQuestImageryStyle.SatelliteMapStyle })
End Sub
End Class
End Namespace
In C#:
using Infragistics.Controls.Maps;
namespace IGGeographicMap.Extensions
{
/// <summary>
/// Represents geographic imagery with street map style from the Map Quest service
/// </summary>
public class MapQuestStreetImagery : GeographicMapImagery
{
public MapQuestStreetImagery()
: base(new MapQuestTileSource { TileMapStyle = MapQuestImageryStyle.StreetMapStyle })
{ }
}
/// <summary>
/// Represents geographic imagery with satellite map style from the Map Quest service
/// </summary>
public class MapQuestSatelliteImagery : GeographicMapImagery
{
public MapQuestSatelliteImagery()
: base(new MapQuestTileSource { TileMapStyle = MapQuestImageryStyle.SatelliteMapStyle })
{ }
}
}
Create an instance of MapQuestStreetImagery object and set it to the Map Background Content of the XamGeographicMap control.
In XAML:
<ig:XamGeographicMap x:Name="GeoMap">
<ig:XamGeographicMap.BackgroundContent>
<custom:MapQuestStreetImagery />
</ig:XamGeographicMap.BackgroundContent>
</ig:XamGeographicMap>
In Visual Basic:
Dim geoImagery = New MapQuestStreetImagery()
Me.GeoMap.BackgroundContent = geoImagery
In C#:
var geoImagery = new MapQuestStreetImagery();
this.GeoMap.BackgroundContent = geoImagery;
Build and run your project to verify the result. If you have implemented the steps correctly, the displayed XamGeographicMap should look like the one in the Preview section above.
The following topics provide additional information related to this topic.