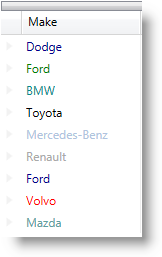
This topic describes how to bind cell settings to data item properties using the CellBindings collection property in the xamDataPresenter™ controls.
The following topics are prerequisites to understanding this topic:
This topic contains the following sections:
Use the CellBindings
collection property to facilitate the binding of a cell settings to properties exposed off the associated data item.
Create a CellBinding
instance for every field’s cell property which you would like to bind to a data item property and add it to the CellBindings
collection.
The following table maps the desired configuration to the property settings that manage it.
The screenshot below demonstrates how a field’s cells would look as a result of the following CellBinding
settings:
Following is the code that implements this example.
In C#:
public class Car : INotifyPropertyChanged
{
private string _make;
public string Make
{
get { return _make; }
set
{
if (_make != value)
{
_make = value;
NotifyPropertyChanged("Make");
}
}
}
private Brush _color;
public Brush Color
{
get { return _color; }
set
{
if (_color != value)
{
_color = value;
NotifyPropertyChanged("Color");
}
}
}
#region INotifyPropertyChanged Members
public event PropertyChangedEventHandler PropertyChanged;
private void NotifyPropertyChanged(String info)
{
if (PropertyChanged != null)
{
PropertyChanged(this, new PropertyChangedEventArgs(info));
}
}
#endregion
}
In XAML:
<igDP:XamDataGrid x:Name="XamDataGrid1"
DataSource="{Binding Path=Cars}">
<igDP:XamDataGrid.FieldLayoutSettings>
<igDP:FieldLayoutSettings AutoGenerateFields="False" />
</igDP:XamDataGrid.FieldLayoutSettings>
<igDP:XamDataGrid.FieldLayouts>
<igDP:FieldLayout>
<igDP:FieldLayout.Fields>
<igDP:TextField Name="Make">
<igDP:TextField.CellBindings>
<igDP:CellBinding Property="Foreground"
Target="CellValuePresenter"
Binding="{Binding Path=DataItem.Color}" />
</igDP:TextField.CellBindings>
</igDP:TextField>
</igDP:FieldLayout.Fields>
</igDP:FieldLayout>
</igDP:XamDataGrid.FieldLayouts>
</igDP:XamDataGrid>
The following topics provide additional information related to this topic.