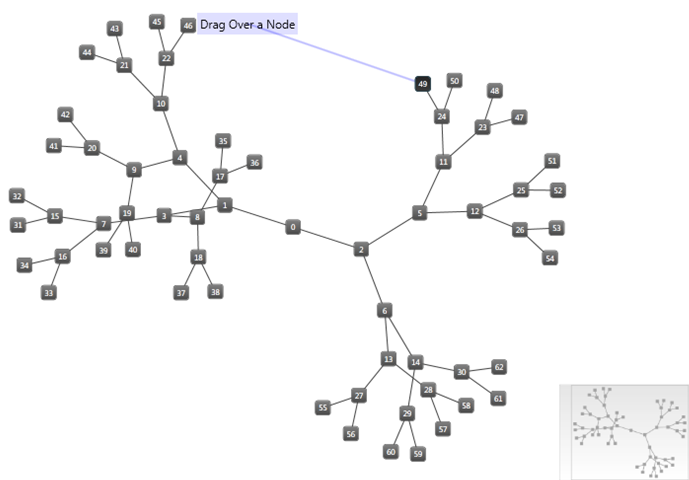
This topic demonstrates how to implement dragging and dropping with NetworkNode and Drag and Drop Framework™.
The topic is organized as follows:
When implementing Drag and Drop the underlying data in the hierarchy must be updated manually. This is a requirement of the xamNetworkNode™ control so it could reflect the changes.
Following is a preview of a node being dragged reorganizing the network structure:
Figure 1: Dragging a node
Required NuGet package references:
Infragistics.WPF.NetworkNode
For more information on setting up the NuGet feed and adding NuGet packages, you can take a look at the following documentation: NuGet Feeds.
Conceptual overview of the procedure:
Add namespace declarations.
Add the XML namespace declaration to your XAML file:
In XAML:
xmlns:ig="http://schemas.infragistics.com/xaml"
Place using/Imports in your code behind:
In Visual Basic:
Imports Infragistics.Controls.Maps
Imports Infragistics.DragDrop
In C#:
using Infragistics.Controls.Maps;
using Infragistics.DragDrop;
Configure the xamNetworkNode as a Drop Target.
In XAML:
<ig:XamNetworkNode>
<ig:DragDropManager.DropTarget>
<ig:DropTarget IsDropTarget="True" />
</ig:DragDropManager.DropTarget>
</ig:XamNetworkNode>
In Visual Basic:
Dim networkNode As New XamNetworkNode()
'Create a new DropTarget object.
Dim dropTarget As New DropTarget()
dropTarget.IsDropTarget = True
'Make the NetworkNode a Drop Target.
DragDropManager.SetDropTarget(networkNode, dropTarget)
In C#:
XamNetworkNode networkNode = new XamNetworkNode();
//Create a new DropTarget object.
DropTarget dropTarget = new DropTarget();
dropTarget.IsDropTarget = true;
//Make the NetworkNode a Drop Target.
DragDropManager.SetDropTarget(networkNode, dropTarget);
Create the Nodes Drag Sources and Drop Targets.
To work with nodes, the xamNetworkNode control uses two key classes:
NetworkNodeNode – its objects are the actual Network nodes. This class carries information about the parent and children nodes, the data displayed by the node, and a reference to the NetworkNodeNodeLayout object used to create the node.
NetworkNodeNodeControl – its objects serve as visual representations of the NetworkNodeNode objects.
Therefore, the Drag Sources and Drop Targets are NetworkNodeNodeControl objects.
Whenever a node is moved out of the visible area of the NetworkNode due to panning, the NetworkNodeNodeControl related to the node is disposed. Also, when the children of a node are collapsed, their NetworkNodeNodeControls are disposed.
If a node has to be shown, a new NetworkNodeNodeControl associated to the node is created and the NodeControlAttachedEvent is raised.
If working with Drag and Drop Framework, the DragSource and DropTarget properties must be attached to the NetworkNodeNodeControl objects when they are created.
Assign an event handler to the NodeControlAttachedEvent.
In XAML:
<ig:XamNetworkNode
NodeControlAttachedEvent="NetworkNode_NodeControlAttachedEvent">
</ig:XamNetworkNode>
Configure the new NetworkNodeNodeControl as a Drag Source and Drop Target.
In Visual Basic:
Private Sub NetworkNode_NodeControlAttachedEvent(sender As Object, e As NetworkNodeNodeEventArgs)
'Create a new DragSource object.
Dim dragSource As New DragSource()
dragSource.IsDraggable = True
'dragSource.DragChannels = assign drag channels
dragSource.Drop += Node_Drop
'Make the Node a Drag Source.
DragDropManager.SetDragSource(e.Node, dragSource)
'Create a new DropTarget object.
Dim dropTarget As New DropTarget()
dropTarget.IsDropTarget = True
'dropTarget.DropChannels = assign drop channels
'Make the Node a Drop Target.
DragDropManager.SetDropTarget(e.Node, dropTarget)
End Sub
In C#:
private void NetworkNode_NodeControlAttachedEvent(object sender, NetworkNodeNodeEventArgs e)
{
//Create a new DragSource object.
DragSource dragSource = new DragSource();
dragSource.IsDraggable = true;
//dragSource.DragChannels = assign drag channels
dragSource.Drop += Node_Drop;
//Make the Node a Drag Source.
DragDropManager.SetDragSource(e.Node, dragSource);
//Create a new DropTarget object.
DropTarget dropTarget = new DropTarget();
dropTarget.IsDropTarget = true;
//dropTarget.DropChannels = assign drop channels
//Make the Node a Drop Target.
DragDropManager.SetDropTarget(e.Node, dropTarget);
}
Handle the Drop event of the Drag Source.
In Visual Basic:
Private Sub Node_Drop(sender As Object, e As DropEventArgs)
'Get the dragged NetworkNodeNodeControl object.
Dim draggedNodeControl As NetworkNodeNodeControl = _
TryCast(e.DragSource, NetworkNodeNodeControl)
'Get the dragged NetworkNodeNode object.
Dim draggedNode As NetworkNodeNode = draggedNodeControl.Node
'Get the underlying data.
Dim data As Object = draggedNode.Data
'TODO: Modify the underlying data.
End Sub
In C#:
private void Node_Drop(object sender, DropEventArgs e)
{
//Get the dragged NetworkNodeNodeControl object.
NetworkNodeNodeControl draggedNodeControl =
e.DragSource as NetworkNodeNodeControl;
//Get the dragged NetworkNodeNode object.
NetworkNodeNode draggedNode = draggedNodeControl.Node;
//Get the underlying data.
object data = draggedNode.Data;
//TODO: Modify the underlying data.
}