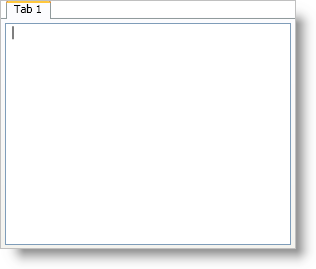
You can add xamTabControl™ to a Window using the same pattern as any control found in Microsoft® Windows® Presentation Foundation. This pattern involves using a layout container as the main content of the Window and then adding a control to the Children collection of the layout container. It is important to name the layout container in XAML so that you can reference it from the code-behind.
You will add xamTabControl to a Window using XAML or procedural code. You will then add a tab to xamTabControl, and set the tab’s content to a RichTextBox control.
When you run the finished project, you should see a xamTabControl in your Window that is similar to the screen shot below.
Create a Microsoft® Windows® Presentation Foundation Window project.
Add the following NuGet package reference to your application:
Infragistics.WPF
For more information on setting up the NuGet feed and adding NuGet packages, you can take a look at the following documentation: NuGet Feeds.
Add an XML namespace declaration for xamTabControl inside the opening Window tag in XAML. In code-behind you will need using/Imports directives so you don’t have to type out a member’s fully qualified name.
In XAML:
xmlns:igWindows="http://infragistics.com/Windows"
In Visual Basic:
Imports Infragistics.Windows.Controls
In C#:
using Infragistics.Windows.Controls;
Name the default Grid layout panel in the Window so that you can reference it in the code-behind.
In XAML:
<Grid Name="layoutRoot"> </Grid>
Create an instance of xamTabControl and name it.
In XAML:
<igWindows:XamTabControl Name="xamTabControl1"> <!-- TODO: Add Tabs here --> </igWindows:XamTabControl>
Create an instance of the xamTabControl control in the Window constructor after the InitializeComponent method and add it to the Grid’s Children collection.
In Visual Basic:
Dim xamTabControl1 As New XamTabControl() layoutRoot.Children.Add(xamTabControl1)
In C#:
XamTabControl xamTabControl1 = new XamTabControl(); layoutRoot.Children.Add(xamTabControl1);
You do not have to explicitly declare tags for xamTabControl’s Items collection.
In XAML:
<igWindows:TabItemEx Header="Tab 1"> <!--TODO: Add content here--></igWindows:TabItemEx>
In Visual Basic:
Dim tab1 As New TabItemEx() tab1.Header = "Tab 1" xamTabControl1.Items.Add(tab1)
In C#:
TabItemEx tab1 = new TabItemEx(); tab1.Header = "Tab 1"; xamTabControl1.Items.Add(tab1);
Add a RichTextBox control to the tab item.
In XAML:
<RichTextBox />
In Visual Basic:
tab1.Content = New RichTextBox()
In C#:
tab1.Content = new RichTextBox();
Run the project.