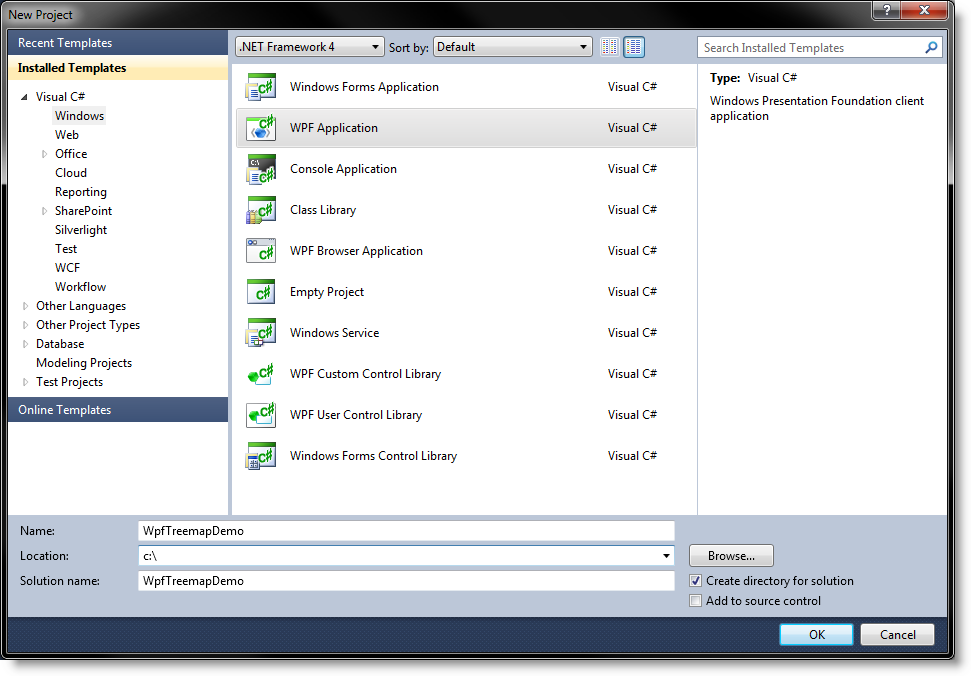
In this article you will learn how to bind the xamTreemap™ control to an ADO.NET Entity Data Model .
Create a new Windows Presentation Foundation Application in Visual Studio 2010 named WpfTreemapDemo.
Add the following NuGet package to your application:
Infragistics.WPF.Treemap
For more information on setting up the NuGet feed and adding NuGet packages, you can take a look at the following documentation: NuGet Feeds.
Right-click on your project and select Add > New Item .
Create a new ADO.NET Entity Data Model named TreemapModel.
Choose your database. For this sample we are going to connect to a sample database – AdventureWorks2008.
Select the tables you need. For this sample we are going to work with ProductCategory, ProductSubcategory and Product.
These tables linked together will produce a hierarchical data source which we will pass to the xamTreemap control. Visual Studio automatically creates navigation properties from which we will use:
ProductCategory.ProductSubcategories
ProductSubcategory.Products
Edit MainWindow.xaml:
In XAML:
<Window x:Class="WpfTreemapDemo.MainWindow" xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation" xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml" xmlns:ig="http://schemas.infragistics.com/xaml" Loaded="MainWindow_Loaded" Title="MainWindow"> <Window.Resources> <!--The data source for the xamTreemap control--> <CollectionViewSource x:Key="ProductCategoriesViewSource" /> </Window.Resources> <Grid DataContext="{StaticResource ProductCategoriesViewSource}"> <ig:XamTreemap x:Name="Treemap" ItemsSource="{Binding}"> <ig:XamTreemap.NodeBinders> <!--Binder for the ProductCategory items--> <ig:NodeBinder TextPath="Name" TargetTypeName="ProductCategory" ItemsSourcePath="ProductSubcategories" /> <!--Binder for the ProductSubcategory items--> <ig:NodeBinder TextPath="Name" TargetTypeName="ProductSubcategory" ItemsSourcePath="Products" /> <!--Binder for the Product items--> <ig:NodeBinder TextPath="Name" ValuePath="StandardCost" TargetTypeName="Product" /> </ig:XamTreemap.NodeBinders> </ig:XamTreemap> </Grid> </Window>
In the code-behind file for MainWindow.xaml add:
In Visual Basic:
Imports System.Data.Objects Class MainWindow Private Sub Window_Loaded _ (ByVal sender As System.Object, ByVal e As System.Windows.RoutedEventArgs) Dim adventureWorks2008Entities As New AdventureWorks2008Entities() 'Get an instance of the CollectionViewSource resource from MainWindow.xaml Dim productCategoriesViewSource As CollectionViewSource = DirectCast(Me.FindResource("ProductCategoriesViewSource"), CollectionViewSource) 'Create a new query for the ProductCategory items Dim productCategoriesQuery As ObjectQuery(Of WpfTreemapDemo.ProductCategory) = adventureWorks2008Entities.ProductCategories 'Execute the query productCategoriesViewSource.Source = productCategoriesQuery.Execute(MergeOption.AppendOnly) End Sub End Class
In C#:
using System.Data.Objects; using System.Windows; using System.Windows.Data; namespace WpfTreemapDemo { public partial class MainWindow : Window { public MainWindow() { InitializeComponent(); } void MainWindow_Loaded(object sender, RoutedEventArgs e) { AdventureWorks2008Entities adventureWorks2008Entities = new AdventureWorks2008Entities(); //Get an instance of the CollectionViewSource resource from MainWindow.xaml CollectionViewSource productCategoriesViewSource = (CollectionViewSource)(this.FindResource("ProductCategoriesViewSource")); //Create a new query for the ProductCategory items ObjectQuery<WpfTreemapDemo.ProductCategory> productCategoriesQuery = adventureWorks2008Entities.ProductCategories; //Execute the query productCategoriesViewSource.Source = productCategoriesQuery.Execute(MergeOption.AppendOnly); } } }
Execute your application.