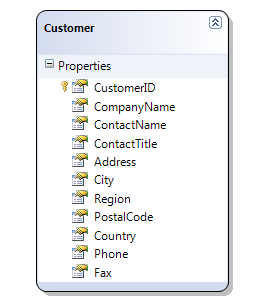
The DomainDataSource control component is fully supported by all Ultimate UI for ASP.NET AJAX controls. You can bind to it directly or use the WebHierarchicalDataSource™ component to obtain hierarchical data. For more information on using the WebHierarchicalDataSource component to bind to data, see Getting Started with WebHierarchicalDataSource.
You will learn how to bind WebDataGrid to the DomainDataSource component using the Northwind Customers table.
Open Visual Studio 2010, create a new ASP.NET Web Application and add a Data Model to it. This tutorial uses the LINQ to SQL model.
Before continuing to the next step you need to build the project so that the data classes and data context are generated and available to be exposed to the Domain Service.
Add a new item to the web application and select the Domain Service Class template under the Web category. Type NorthwindDomainService for name and click Add.
From the Domain Service dialogue window select NorthwindDataContext and choose Customer entity. Click the Enable editing check box.
By clicking OK the domain service will generate the following code into the NorthwindDomainService class:
In C#:
public class NorthwindDomainService : LinqToSqlDomainService<NorthwindDataContext> { public IQueryable<Customer> GetCustomers() { return this.DataContext.Customers; } public void InsertCustomer(Customer customer) { this.DataContext.Customers.InsertOnSubmit(customer); } public void UpdateCustomer(Customer currentCustomer) { this.DataContext.Customers.Attach(currentCustomer, this.ChangeSet.GetOriginal(currentCustomer)); } public void DeleteCustomer(Customer customer) { this.DataContext.Customers.Attach(customer); this.DataContext.Customers.DeleteOnSubmit(customer); } }
At your aspx page, drag the DomainDataSource component from the Toolbox. From Design, right-click on DomainDataSource and then select Configure Data Source.
The following markup appears for DomainDataSource component.
In HTML:
<asp:DomainDataSource ID="DomainDataSource1" runat="server" DomainServiceTypeName="DomainDataSourceApplication.NorthwindDomainService" QueryName="GetCustomers"> </asp:DomainDataSource>
Drag WebScriptManager and WebDataGrid from the toolbox.
Set the WebDataGrid’s DataSourceID property to DomainDataSource1 and DataKeyFields to CustomerID.
Run the application. WebDataGrid binds to the Customers table and displays its data.