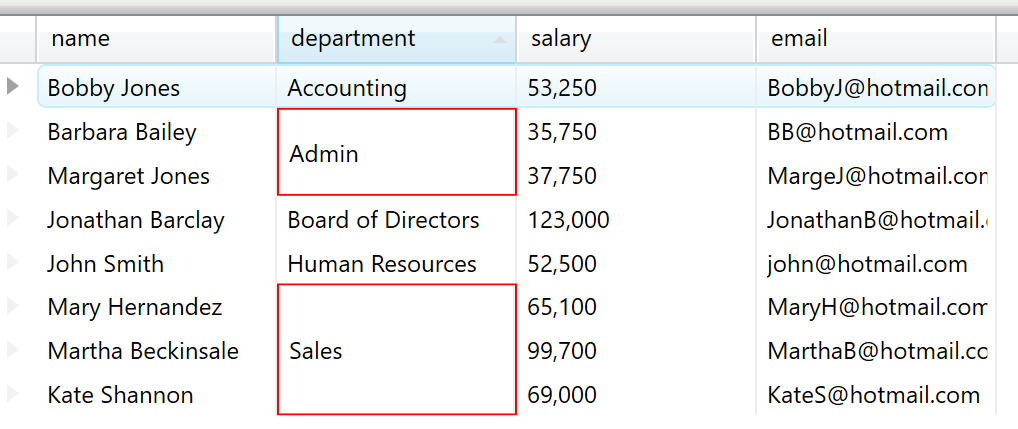
This topic gives an overview of the xamDataGrid control’s grouping functionality allowing users to group data into more readable and navigable arrangements.
The DataPresenter controls have several modes and properties for this feature, which you can configure via the FieldSettings object.
The following topic is a prerequisite to understanding this topic:
This topic contains the following sections:
A DataPresenter control such as a XamDataGrid control may opt-in and detect when adjacent sibling DataRecords for a specific Field contains the same value. While the cells are not in edit mode, the value displays across the DataRecords, and is represented as by a MergedCellPresenter.
The xamDataGrid is highly user configurable using these settings.
You can set this MergedCellMode
property to a enum of type MergedCellMode to specify if and how cell merging is performed.
This property can be set to a custom implementation of the IMergedCellEvaluator interface. The interface is used to specify custom logic for determining which cells get merged.
You can set this MergedCellEvaluationCriteria
property to a enum of type MergedCellEvaluationCriteria to determine which cells can be merged based on either the cell’s value or its display text.
The following example code demonstrates how to merge cells for all fields.
In XAML:
<igDP:XamDataPresenter Name="xamDataPresenter1" BindToSampleData="True"> <igDP:XamDataGrid.FieldSettings> <igDP:FieldSettings MergedCellMode="Always" > </igDP:FieldSettings> </igDP:XamDataGrid.FieldSettings> </igDP:XamDataPresenter>
In C#:
using Infragistics.Windows.DataPresenter; ... this.xamDataPresenter1.FieldSettings.MergedCellMode = MergedCellMode.Always; ...
In Visual Basic:
Imports Infragistics.Windows.DataPresenter ... Me.xamDataPresenter1.FieldSettings.MergedCellMode = MergedCellMode.Always ...
The following screenshot is an example of using the code above.
The following example code demonstrates how to merge cells for a specific field.
In XAML:
<igDP:XamDataGrid DataSource="{Binding}" > <igDP:XamDataGrid.FieldLayouts> <igDP:FieldLayout Key="layout1"> <igDP:FieldLayout.Fields> <igDP:Field x:Name="department"> <igDP:Field.Settings> <igDP:FieldSettings MergedCellMode="Always" > </igDP:FieldSettings> </igDP:Field> </igDP:FieldLayout.Fields> </igDP:FieldLayout> </igDP:XamDataGrid.FieldLayouts> </igDP:XamDataGrid>
In C#:
using Infragistics.Windows.DataPresenter; ... this.xamDataPresenter1.FieldLayouts["layout1"].Fields["department"].Settings.MergedCellMode = Infragistics.Windows.DataPresenter.MergedCellMode.Always; ...
In Visual Basic:
Imports Infragistics.Windows.DataPresenter ... Me.xamDataPresenter1.FieldLayouts("layout1").Fields("department").Settings.MergedCellMode = Infragistics.Windows.DataPresenter.MergedCellMode.Always ...