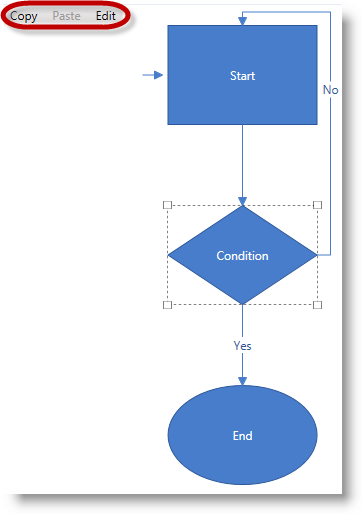
This topic explains how to use the commands provided by xamDiagram™ in UI elements such as menus and buttons. For a list of the pre-configured keyboard shortcuts associated with the basic commands, see Configuring Keyboard Shortcuts.
The following topics are prerequisites to understanding this topic:
This topic contains the following sections:
The xamDiagram control supports commands for performing common user operations on its items such as Copy/Paste, Select All, etc., as well as for item editing and showing/hiding its Item Options pane. By default, most of these commands are associated with keyboard shortcuts (see the Configuring Keyboard Shortcuts for details), but they can also be configured to be invoked by a user interaction with another control, e.g. Menu , Button , etc..
The basic diagram operations (Copy, Paste, Delete, Cut, Select All, Undo, Redo) are implemented in the API as ApplicationCommands
because the effect of their invoking resembles the expected behavior of any other UI element which may be present in the application. There are also a few commands, such as the ShowOptionsPane, EnterEditMode, and CloseOptionsPane, which carry diagram-specific logic and have been implemented as members of the DiagramCommands class. This separation is reflected in the different ways of accessing the commands from the two groups.
The actions associated with the different commands are applied to the diagram or to any of its items when the control owns the focus and an interaction which invokes that command occurs.
The following table summarizes the commands supported by the xamDiagram control and maps them to the pre-configured keyboard shortcuts.
This procedure walks you through the process of configuring UI elements with some xamDiagram commands. For the sake of example, in this procedure, a Menu control is used and its three MenuItems configured to triggers Copy, Paste, and EnterEditMode commands, respectively, on xamDiagram ’s items.
The following screenshot demonstrates a menu with Copy, Paste, and Edit options added to the diagram added as a result of this procedure.
To complete the procedure, you need the following:
A Microsoft® Visual Studio® WPF application with a page.
The required assembly references and namespaces added to the project (For details, see Adding xamDiagram to a Page.)
A xamDiagram control added to the page. (For details, see Adding xamDiagram to a Page.)
Following is a conceptual overview of the process:
1. Adding the Menu control
2. Configuring the MenuItem objects interaction commands
3. (Optional) Verifying the result of the procedure
The following steps demonstrate how to configure the MenuItems
of a Menu control to invoke xamDiagram commands.
Create an instance of the Menu control and add it to the page.
In XAML:
<Menu VerticalAlignment="Top">
</Menu>
1. Configure the Copy and Paste menu items.
Because the copy and paste commands for the diagram are ApplicationCommands
, configure the Command
parameter of the respective MenuItem
to perform copy or paste action . The action will be applied on the item of the diagram that has the focus (and to any other UI element on the page, for that matter, if it has the focus).
In XAML:
<MenuItem Command="ApplicationCommands.Copy" />
<MenuItem Command="ApplicationCommands.Paste"/>
2. Configure the Edit menu item.
Because the EnterEditMode
command belongs to the DiagramCommands
class, to bind it to a specific MenuItem
, set the Command
and CommandTarget
properties of the MenuItem
object . The latter setting is used to specify that the command binding target is the diagram object.
In XAML:
<MenuItem Header="Edit"
Command="{x:Static ig:DiagramCommands.EnterEditMode}"
CommandTarget="{Binding ElementName=diagram}"/>
3. Add the menu items to the menu.
In XAML:
<Menu VerticalAlignment="Top">
<MenuItem Command="ApplicationCommands.Copy" />
<MenuItem Command="ApplicationCommands.Paste" />
<MenuItem Header="Edit"
Command="{x:Static ig:DiagramCommands.EnterEditMode}"
CommandTarget="{Binding ElementName=diagram}"/>
</Menu>
As a result of this procedure you should have a functional menu like the one demonstrated in the Preview.
You can test the functionality of the menu items, by, for example, selecting a node, clicking Copy from the menu and then pasting it again using the Paste menu item on a location in the diagram space.
Following is the full code for this procedure.
In XAML:
<Grid>
<Grid.RowDefinitions>
<RowDefinition Height="auto" />
<RowDefinition Height="5*" />
</Grid.RowDefinitions>
<Menu VerticalAlignment="Top">
<MenuItem Command="ApplicationCommands.Copy" />
<MenuItem Command="ApplicationCommands.Paste" />
<MenuItem Header="Edit"
Command="{x:Static ig:DiagramCommands.EnterEditMode}"
CommandTarget="{Binding ElementName=diagram}" />
</Menu>
<ig:XamDiagram x:Name="diagram" Grid.Row="1">
<ig:DiagramNode Key="node1"
Content="Start"
Height="100"
Width="150"
Position="200,20" />
<ig:DiagramNode Key="node2"
Content="Condition"
Height="100"
Width="100"
ShapeType="Rhombus"
Position="225,200"
MaintainAspectRatio="True" />
<ig:DiagramNode Key="node3"
Content="End"
Height="100"
Width="150"
ShapeType="Ellipse"
Position="200,380" />
<ig:DiagramConnection Name="conn12"
StartNodeKey="node1"
EndNodeKey="node2"
ConnectionType="Straight" />
<ig:DiagramConnection Name="conn23"
StartNodeKey="node2"
EndNodeKey="node3"
ConnectionType="Straight"
Content="Yes" />
<ig:DiagramConnection Name="conn21"
StartNodeKey="node2"
EndNodeKey="node1"
StartNodeConnectionPointName="Right"
EndNodeConnectionPointName="Top"
Content="No" />
<ig:DiagramConnection Name="connStart"
StartPosition="175,70"
EndPosition="195,70" />
</ig:XamDiagram>
</Grid>
The following topics provide additional information related to this topic.