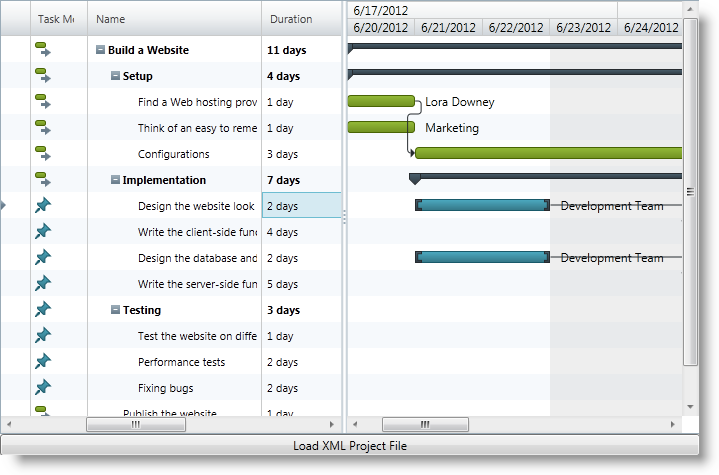
This topic describes how an already created project plan saved in a Microsoft Project™ 2010 XML file is loaded in the xamGantt™ control.
The following topics are prerequisites to understanding this topic:
This topic contains the following sections:
The procedure describes how you can load a MS Project 2010 XML file in xamGantt control.
The following screenshot previews the result.
Following is a conceptual overview of the process:
The following steps demonstrate how to load an XML project file in the xamGantt.
Add a xamGantt control in XAML
Add the xamGantt control to the first row of a Grid container:
In XAML:
<Grid>
<Grid.RowDefinitions>
<RowDefinition Height="*" />
<RowDefinition Height="22" />
</Grid.RowDefinitions>
<ig:XamGantt x:Name="gantt" />
<!-- Add other controls here -->
</Grid>
Add a Button to open a dialog to browse for project XML file
Add a Button control in the second row of the Grid container:
In XAML:
<Button x:Name="Btn_LoadProject"
Grid.Row="1"
Content="Load XML Project File"
Click="Btn_LoadProject_Click"/>
Add required references in code-behind
Add the following using and Imports declarations in the code-behind:
In C#:
using System;
using System.IO;
using System.Windows;
using Infragistics;
using Infragistics.Controls.Schedules;
In Visual Basic:
Imports System.IO
Imports Infragistics
Imports Infragistics.Controls.Schedules
Imports Microsoft.Win32
Handle the Button Click event
Handle the Button Click event to open an XML project file.
For more information, see the Code Example: Handling the Button Click event to browse an XML file.
Load project in the xamGantt
Use the Project LoadFromProjectXml method to load a project from a stream.
For more information, see the Code Example: Loading a Project to the xamGantt Control.
The following table lists the code examples included in this topic.
The example code below illustrates how to find, open and transform an XML project file to a Stream object.
In C#:
using Microsoft.Win32;
private void Btn_LoadProject_Click(object sender, RoutedEventArgs e)
{
OpenFileDialog dialog = new OpenFileDialog();
dialog.Multiselect = false;
dialog.InitialDirectory = "c:\\";
dialog.Filter = "MS Project XML (.xml)|*.xml|All Files (*.*)|*.* ";
bool? isOpened = dialog.ShowDialog();
if (isOpened == true)
{
try
{
using (Stream stream = dialog.OpenFile())
{
LoadProjectFromStream(stream);
stream.Close();
}
}
catch (Exception ex)
{
MessageBox.Show(ex.Message);
}
}
}
In Visual Basic:
Imports Microsoft.Win32
Private Sub Btn_LoadProject_Click(sender As Object, e As RoutedEventArgs)
Dim dialog As New OpenFileDialog()
dialog.Multiselect = False
dialog.InitialDirectory = "c:\"
dialog.Filter = "MS Project XML (.xml)|*.xml|All Files (*.*)|*.* "
Dim isOpened As System.Nullable(Of Boolean) = dialog.ShowDialog()
If isOpened = True Then
Try
Using stream As Stream = dialog.OpenFile()
LoadProjectFromStream(stream)
stream.Close()
End Using
Catch ex As Exception
MessageBox.Show(ex.Message)
End Try
End If
End Sub
The example code below, illustrates how to load a project from the Stream object created in the previous code example.
The Project LoadFromProjectXml method takes a Stream as a parameter.
In C#:
private void LoadProjectFromStream(Stream stream)
{
// Create a xamGantt Project and load project data from the resulting Stream
var project = new Project();
// Load a project from stream
project.LoadFromProjectXml(stream);
this.gantt.VisibleDateRange = new DateRange(project.Start, project.Finish);
this.gantt.Project = project;
}
In Visual Basic:
Private Sub LoadProjectFromStream(stream As Stream)
' Create a xamGantt Project and load project data from a Stream
Dim project = New Project()
' Load a project from stream
project.LoadFromProjectXml(stream)
Me.gantt.VisibleDateRange = New DateRange(project.Start, project.Finish)
Me.gantt.Project = project
End Sub
The following topics provide additional information related to this topic.