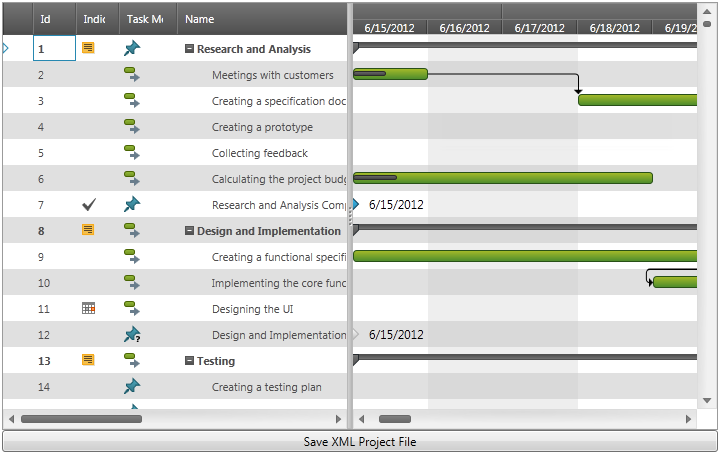
This topic explains how to save a xamGantt ™ project plan in a Microsoft Project XML file.
The following topic is a prerequisite to understanding this topic:
This topic contains the following sections:
The procedure describes how to save a xamGantt project plan to an MS Project XML file.
The following screenshot is a preview of the results.
Following is a conceptual overview of the process:
Add a xamGantt control in XAML
Add an open dialog box button for saving the project plan as XML file
Add required references in code-behind
Handle the button click event
Save the project plan as MS Project XML file
The following steps demonstrate saving a xamGantt project plan to an XML file.
Add a xamGantt control in XAML
Add the xamGantt control to the first row of a Grid container:
In XAML:
<Grid>
<Grid.RowDefinitions>
<RowDefinition Height="*" />
<RowDefinition Height="Auto" />
</Grid.RowDefinitions>
<ig:XamGantt x:Name="gantt" />
<!-- Add other controls here -->
</Grid>
Add a button for showing the save to XML file dialog
Add a Button control in the second row of the Grid container:
In XAML:
<Button x:Name="Btn_SaveProject"
Grid.Row="1"
Content="Save XML Project File"
Click="Btn_SaveProject_Click"/>
Add required references in code-behind
Add the following using or Imports declarations in the code-behind:
In C#:
using System;
using System.IO;
using System.Windows;
using Infragistics;
using Infragistics.Controls.Schedules;
using Microsoft.Win32;
In Visual Basic:
Imports System.IO
Imports Infragistics
Imports Infragistics.Controls.Schedules
Imports Microsoft.Win32
Handle the button click event
Handle the button click event to save the xamGantt project plan to an MS Project XML file.
For more information, see the Code Example: Handle the Button Click Event to Save the Project Plan as XML File
Save the xamGantt project to an MS Project XML file
Use the Project’s SaveAsProjectXml method to save a project plan to a stream.
For more information, see the Code Example: Saving a xamGantt’s Project Plan to an MS Project XML File
The following table lists the code examples included in this topic.
The example code uses the SaveFileDialog class to save a xamGantt’s project plan to an MS Project XML file.
In C#:
using Microsoft.Win32;
private void Btn_SaveProject_Click(object sender, RoutedEventArgs e)
{
SaveFileDialog dialog = new SaveFileDialog();
dialog.InitialDirectory = "c:\\";
dialog.Filter = "MS Project XML (.xml)|*.xml|All Files (*.*)|*.* ";
bool? isSaved = dialog.ShowDialog();
if (isSaved == true)
{
try
{
using (Stream stream = dialog.OpenFile())
{
SaveProjectToStream(stream);
stream.Close();
}
}
catch (Exception ex)
{
MessageBox.Show(ex.Message);
}
}
}
In Visual Basic:
Imports Microsoft.Win32
Private Sub Btn_SaveProject_Click(sender As Object, e As RoutedEventArgs)
Dim dialog As New SaveFileDialog()
dialog.InitialDirectory = "c:\"
dialog.Filter = "MS Project XML (.xml)|*.xml|All Files (*.*)|*.* "
Dim isSaved As System.Nullable(Of Boolean) = dialog.ShowDialog()
If isSaved = True Then
Try
Using stream As Stream = dialog.OpenFile()
SaveProjectToStream(stream)
stream.Close()
End Using
Catch ex As Exception
MessageBox.Show(ex.Message)
End Try
End If
End Sub
The following code examples demonstrate how to save xamGantt project plans to an MS Project XML file in both C# and Visual Basic.
In C#:
private void SaveProjectToStream(Stream stream)
{
// obtain the xamGantt Project
var project = this.gantt.Project;
project.SaveAsProjectXml(stream);
}
In Visual Basic:
Private Sub SaveProjectToStream(stream As Stream)
' obtain the xamGantt Project
Dim project = Me.gantt.Project
project.SaveAsProjectXml(stream)
End Sub
The following topics provide additional information related to this topic.