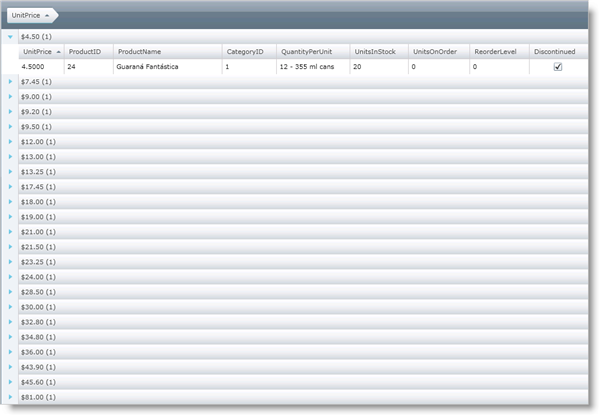
Please note that this control has been retired and is now obsolete to the XamDataGrid control, and as such, we recommend migrating to that control. It will not be receiving any new features, bug fixes, or support going forward. For help or questions on migrating your codebase to the XamDataGrid, please contact support.
The GroupBy feature of xamGrid groups data into GroupByRow objects. These objects have headers that display the common values of the rows grouped within, by default. You can change the caption to display custom information.
A column in xamGrid provides a GroupByItemTemplate property that you can use to set up a data template to display custom content for the GroupByRow objects. The data context of the data template is of type GroupByDataContext which has three properties available:
The following code shows you how to display the value, formatted as currency, and the number of grouped rows for each GroupByRow objects in xamGrid. The example uses a value converter to format the text the GroupBy Row displays.
In XAML:
<UserControl.Resources> <local:DataUtil x:Key="DataUtil" /> <helper:GroupByValueConverter x:Key="GroupByValueConverter" /> </UserControl.Resources> <Grid x:Name="LayoutRoot" Background="White"> <ig:XamGrid x:Name="xamGrid1" ItemsSource="{Binding Source={StaticResource DataUtil}, Path=Products}"> <ig:XamGrid.GroupBySettings> <ig:GroupBySettings AllowGroupByArea="Top" /> </ig:XamGrid.GroupBySettings> <ig:XamGrid.Columns> <ig:TextColumn Key="UnitPrice"> <!-- Use data template to set custom text --> <ig:TextColumn.GroupByItemTemplate> <DataTemplate> <StackPanel Orientation="Horizontal"> <!-- Use a value converter to format Value as currency --> <TextBlock Text="{Binding Value, Converter={StaticResource GroupByValueConverter}}"></TextBlock> <TextBlock Text=" (" /> <TextBlock Text="{Binding Count}" /> <TextBlock Text=")" /> </StackPanel> </DataTemplate> </ig:TextColumn.GroupByItemTemplate> </ig:TextColumn> </ig:XamGrid.Columns> </ig:XamGrid> </Grid>
In Visual Basic:
Public Class GroupByValueConverter Implements IValueConverter Public Function Convert(ByVal value As Object, ByVal targetType As System.Type, ByVal parameter As Object, ByVal culture As System.Globalization.CultureInfo) As Object Implements System.Windows.Data.IValueConverter.Convert Return String.Format("{0:C}", value) End Function Public Function ConvertBack(ByVal value As Object, ByVal targetType As System.Type, ByVal parameter As Object, ByVal culture As System.Globalization.CultureInfo) As Object Implements System.Windows.Data.IValueConverter.ConvertBack Throw New NotImplementedException() End Function End Class
In C#:
public class GroupByValueConverter : IValueConverter { #region IValueConverter Members public object Convert(object value, Type targetType, object parameter, System.Globalization.CultureInfo culture) { return string.Format("{0:C}", value); } public object ConvertBack(object value, Type targetType, object parameter, System.Globalization.CultureInfo culture) { throw new NotImplementedException(); } #endregion }