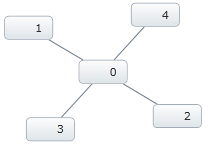
The purpose of this topic is to show how to display the different visual states of nodes in the xamNetworkNode™ control. The visual states are organized in groups, each consisting of several visual state properties where the control can be set to one of the states from any of the selected groups.
Introducing visual states in groups for XamNetworkNode
Common Group
Normal – Not selected nor in focus
Disabled – Gray foreground color and cannot be selected with mouse, Mouse-Over or Mouse-Pressed
Focus group
Focused – Shows with dark color border
Unfocused – Without dark color border
Selection Group
Selected – Shows with dark highlighted background
Unselected – Without dark highlighted background
Editing Group
Editing – Shows with highlighted border
NotEditing – Without highlighted border
The table below lists the required background you need for fully understanding the information in this topic.
The table below lists the configurable visual states of the nodes on the xamNetworkNode control.
The table below maps the various visual states to the corresponding property settings. The properties are accessed through NetworkNodeNode object of XamNetworkNode control. The method Focus() is called on NetworkNodeNode.Control object of XamNetworkNode .
The picture bellow demonstrates the result of normal and disabled states:
The picture bellow demonstrates the result of placing a node in a focused state. The node transitions to an unfocused state when clicking away from the focused node. There is no property or method to specifically unfocus the node. The normal state is considered unfocused, or the node can be unselected to be out of focus.
The picture bellow demonstrates the result of selected state. Unselected state is the same as normal, described above:
The picture bellow demonstrates the result of editing state. Not editing state is the same as normal, described above:
Visual states of various groups defined through code examples.
The following table lists the code examples provided below.
The Disabled state of the node displays with gray foreground color, and will not respond to mouse click, mouse over, or mouse pressed actions.
In C#:
foreach (NetworkNodeNode node in xnn.Nodes) { node.IsEnabled = false; }
In Visual Basic:
For Each node As NetworkNodeNode In xnn.Nodes node.IsEnabled = False Next
The Focused state of the node by default displays with dark color border. If the node is disabled it cannot be in focus. Only one node can be in focus at a time. In this example the node gives a focus to a node using an index.
In C#:
xnn.Nodes.ElementAt(3).Control.Focus();
In Visual Basic:
xnn.Nodes.ElementAt(3).Control.Focus()
The Selected state of the node by default displays with darker background to stand out among other nodes. The selected nodes are added in the SelectedNodes collection of XamNetworkNode.
In C#:
foreach( var node in xnn.Search((NodeModel item) => item.Label.Equals("1"))) { node.IsSelected = true; // Or xnn.SelectedNodes.Add(node); }
In Visual Basic:
For Each node As var In xnn.Search(Function(item As NodeModel) item.Label.Equals("1")) node.IsSelected = True ' Or xnn.SelectedNodes.Add(node) Next
The Editing state of the node is only a visual state; the nodes are not editable. By default the Editing visual state displays with golden color border around the node, while not editing state is the same as normal
In C#:
foreach( var node in xnn.Search((NodeModel item) => item.Label.Equals("1"))) { node.IsEditing = true; }
In Visual Basic:
For Each node As var In xnn.Search(Function(item As NodeModel) item.Label.Equals("1")) node.IsEditing = True Next